What do you do when you want to hang your stockings up on the fireplace but you don’t have one? The answer is simple, you build one! This is what was asked of me by my sister as she wanted a fireplace to decorate her collage apartment.
This project started off will a roll of paper bricks and the idea to use a cardboard box to make a fireplace. Using a large, flat box I first started by cutting out an opening for where the fire would reside. After the opening was cut, I started to lay the brick sheet onto the box securing it using hot glue.
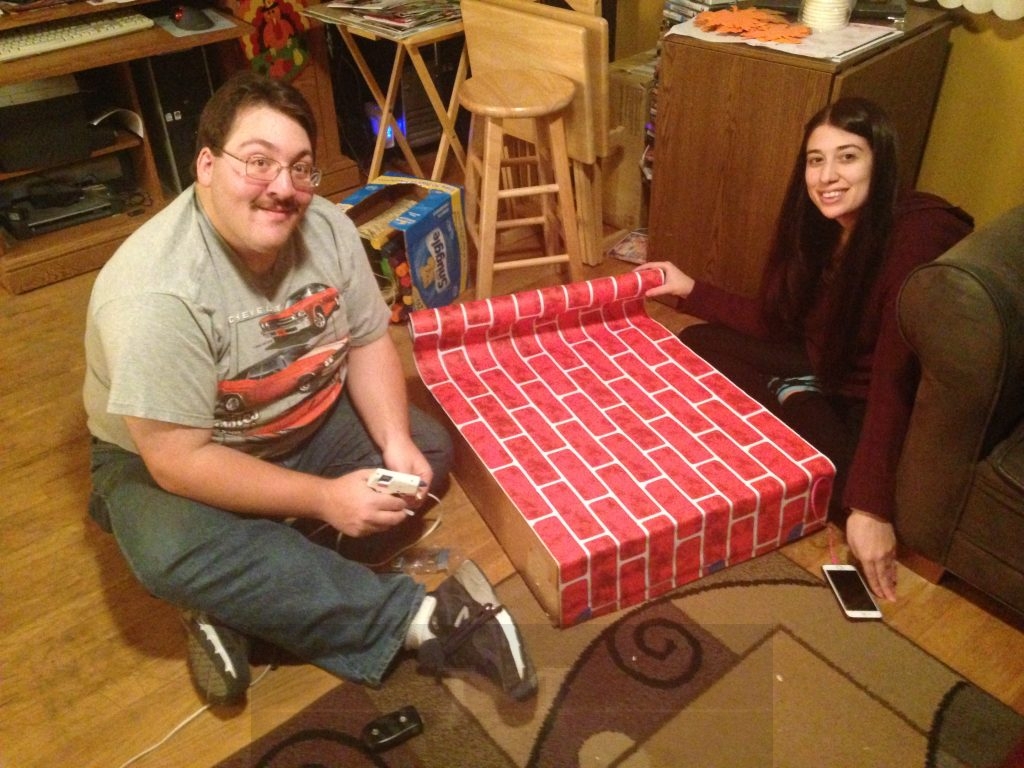
Once the box was wrapped in the paper, I cut the paper around two inches in from the inside of the box opening. I then cut each corner at a 45 degree angle allowing each side to be independent from each other. Then using more hot glue I folded the paper around the inside lip of the box for a nice clean look.
With the opening cut I used the rest of the paper brick to line the inside of the box. This would give the look of a chimney.
Using the cardboard left over from the opening, I drew out a fire grate and then cut it out. Once I was happy with how it looked I then attached black paper using hot glue and cut the paper to fit the cardboard. When that was done I had the basic setup needed for a fireplace, but it was still missing the more important (to me) part. The fire.
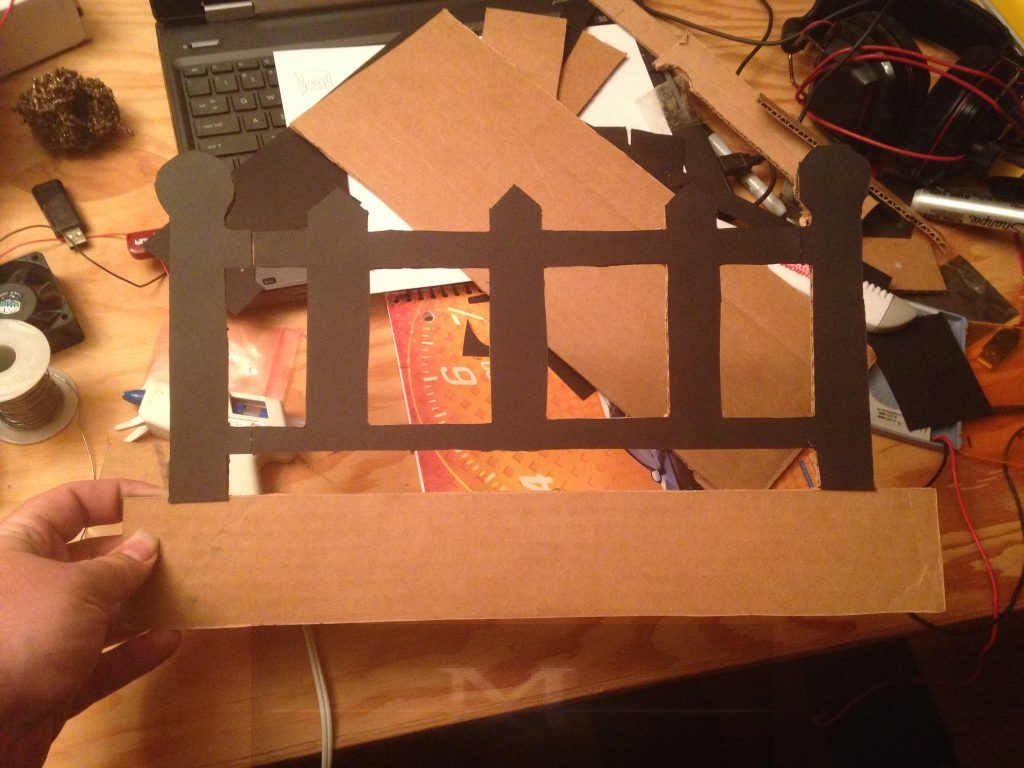
For the fire I decided to use a Adafruit Trinket and a NeoPixel ring to give off a fire flickering effect. I started off getting a 9 volt wall power adapter I hand laying around and a DC to DC 5v converter. The converter was use in order to avoid taxing the Trinket’s on board voltage regulator in driving the NeoPixel ring. This was mounted inside of a project box along with the Trinket and the NeoPixel ring on top. I did this so I would have a fully contained unit that could be easily removed from the fireplace latter.
With the fire part of the fireplace taken care of, all that was left was to put in all together. I mounted the project box to the inside on the fireplace with some hot glue and made a whole in the bottom corner of the box for the power wire to come though. I then secured the fire grate to the rest of the box also with hot glue. Then the final part of the project from me was some paper, to help defuses the light and help give it a glowing ember look. After I was done with assembly my sister fished off the fireplace with some silver garland and fluff to make a mantle.
Now my sister is able to have a fireplace in her apartment for Christmas and can be stowed away the rest of the year.
Here is the Code:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 98 99 100 101 102 103 104 105 106 107 108 109 110 111 112 113 114 115 116 117 118 119 120 121 122 123 124 125 126 127 128 129 130 131 132 133 134 135 136 137 138 139 140 141 142 143 144 145 146 147 148 149 150 151 152 153 154 155 156 157 158 159 160 161 162 163 164 165 166 167 168 169 170 171 172 173 174 175 176 177 178 179 180 181 182 183 184 185 186 187 188 189 |
/** * Arduino Uno - NeoPixel Fire * v. 1.0 * Copyright (C) 2015 Robert Ulbricht * * This program is free software: you can redistribute it and/or modify * it under the terms of the GNU General Public License as published by * the Free Software Foundation, either version 3 of the License, or * (at your option) any later version. * * This program is distributed in the hope that it will be useful, * but WITHOUT ANY WARRANTY; without even the implied warranty of * MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the * GNU General Public License for more details. * * You should have received a copy of the GNU General Public License * along with this program. If not, see <http://www.gnu.org/licenses/>. * https://github.com/RoboUlbricht/arduinoslovakia/blob/master/neopixel/neopixel_fire01/neopixel_fire01.ino * * Modified by Mario Avenoso to work on the Adafruit Trinket * */ #include <Adafruit_NeoPixel.h> #ifdef __AVR__ #include <avr/power.h> #endif // data pin #define PIN 0 // led count #define CNT 36 // Parameter 1 = number of pixels in strip // Parameter 2 = pin number (most are valid) // Parameter 3 = pixel type flags, add together as needed: // NEO_RGB Pixels are wired for RGB bitstream // NEO_GRB Pixels are wired for GRB bitstream // NEO_KHZ400 400 KHz bitstream (e.g. FLORA pixels) // NEO_KHZ800 800 KHz bitstream (e.g. High Density LED strip) Adafruit_NeoPixel strip = Adafruit_NeoPixel(CNT, PIN, NEO_GRB + NEO_KHZ800); uint32_t fire_color = strip.Color ( 80, 35, 00); uint32_t off_color = strip.Color ( 0, 0, 0); /// /// Fire simulator /// class NeoFire { Adafruit_NeoPixel &strip; public: NeoFire(Adafruit_NeoPixel&); void Draw(); void Clear(); void AddColor(uint8_t position, uint32_t color); void SubstractColor(uint8_t position, uint32_t color); uint32_t Blend(uint32_t color1, uint32_t color2); uint32_t Substract(uint32_t color1, uint32_t color2); }; /// /// Constructor /// NeoFire::NeoFire(Adafruit_NeoPixel& n_strip) : strip (n_strip) { } /// /// Set all colors /// void NeoFire::Draw() { Clear(); for(int i=0;i<CNT;i++) { AddColor(i, fire_color); int r = random(80); uint32_t diff_color = strip.Color ( r, r/2, r/2); SubstractColor(i, diff_color); } strip.show(); } /// /// Set color of LED /// void NeoFire::AddColor(uint8_t position, uint32_t color) { uint32_t blended_color = Blend(strip.getPixelColor(position), color); strip.setPixelColor(position, blended_color); } /// /// Set color of LED /// void NeoFire::SubstractColor(uint8_t position, uint32_t color) { uint32_t blended_color = Substract(strip.getPixelColor(position), color); strip.setPixelColor(position, blended_color); } /// /// Color blending /// uint32_t NeoFire::Blend(uint32_t color1, uint32_t color2) { uint8_t r1,g1,b1; uint8_t r2,g2,b2; uint8_t r3,g3,b3; r1 = (uint8_t)(color1 >> 16), g1 = (uint8_t)(color1 >> 8), b1 = (uint8_t)(color1 >> 0); r2 = (uint8_t)(color2 >> 16), g2 = (uint8_t)(color2 >> 8), b2 = (uint8_t)(color2 >> 0); return strip.Color(constrain(r1+r2, 0, 255), constrain(g1+g2, 0, 255), constrain(b1+b2, 0, 255)); } /// /// Color blending /// uint32_t NeoFire::Substract(uint32_t color1, uint32_t color2) { uint8_t r1,g1,b1; uint8_t r2,g2,b2; uint8_t r3,g3,b3; int16_t r,g,b; r1 = (uint8_t)(color1 >> 16), g1 = (uint8_t)(color1 >> 8), b1 = (uint8_t)(color1 >> 0); r2 = (uint8_t)(color2 >> 16), g2 = (uint8_t)(color2 >> 8), b2 = (uint8_t)(color2 >> 0); r=(int16_t)r1-(int16_t)r2; g=(int16_t)g1-(int16_t)g2; b=(int16_t)b1-(int16_t)b2; if(r<0) r=0; if(g<0) g=0; if(b<0) b=0; return strip.Color(r, g, b); } /// /// Every LED to black /// void NeoFire::Clear() { for(uint16_t i=0; i<strip.numPixels (); i++) strip.setPixelColor(i, off_color); } NeoFire fire(strip); /// /// Setup /// void setup() { // This is for Trinket 5V 16MHz, you can remove these three lines if you are not using a Trinket #if defined (__AVR_ATtiny85__) if (F_CPU == 16000000) clock_prescale_set(clock_div_1); #endif // End of trinket special code strip.begin(); strip.show(); // Initialize all pixels to 'off' } /// /// Main loop /// void loop() { fire.Draw(); delay(random(50,150)); } |